ESLint, Prettier란 무엇이고 어떻게 사용할 수 있을까?
개발자들은 각자의 개발 스타일을 갖고 있다. (들여쓰기의 정도, async/await 사용 여부 등...)
많은 코드 스타일들이 프로젝트 한곳에서 모이면 코드가 제각각이 되어 어플리케이션 품질이 저하될 수 있다.
이를 해결하기 위해서 Linter/Formatter를 사용하여 코드 컨벤션 통일을 강제화한다.
대표적인 Linter인 ESLint의 공식 문서에는 아래와 같이 정의한다.
ESLint is an open source project that helps you find and fix problems with your JavaScript code. It doesn't matter if you're writing JavaScript in the browser or on the server, with or without a framework, ESLint can help your code live its best life.
Find issues
ESLint statically analyzes your code to quickly find problems. ESLint is built into most text editors and you can run ESLint as part of your continuous integration pipeline.
Fix problems automatically
Many problems ESLint finds can be automatically fixed. ESLint fixes are syntax-aware so you won't experience errors introduced by traditional find-and-replace algorithms.
Configure everything
Preprocess code, use custom parsers, and write your own rules that work alongside ESLint's built-in rules. Customize ESLint to work exactly the way you need it for your project.
https://eslint.org/
즉 ESLint를 사용하면, ESLint가 문법적인 이슈를 감지하여 심지어 코드를 자동으로 고쳐줄 수 있다는 것이다.
얼마나 장점이 있는지 확인해보기 위해 ESLint를 사용해보자.
원하는 폴더로 이동하여 package.json 파일을 생성한다.
$ npm init -y
엉망인 코드를 작성해본다.
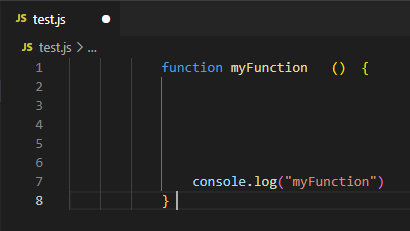
보기에 나쁜 코드를 작성했기 때문에 이런 코드가 운영환경으로 배포된다면 품질이 저하될 수 있다.
ESLint를 통해 문제를 해결해보자.
아래의 커맨드를 통해 .eslintrc.js 파일을 생성해준다. 파일을 통해 ESLint의 config를 관리할 수 있다.
$ npm init @eslint/config
커맨드 수행을 위해서 @eslint/config 패키지 설치가 필요하다고 한다. y를 누르자.

ESLint를 어떤 용도로 사용할 것이냐고 묻는다. 3번째를 선택하자.
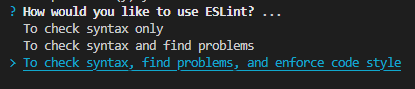
모듈을 어떤 식으로 관리하냐고 묻는다. 용도에 맞춰 선택하자. 나의 경우에는 첫 번째를 선택했다.
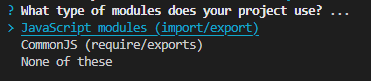
어떤 프레임워크를 사용하고 있냐고 묻는다. 나의 경우에는 React를 선택했다.
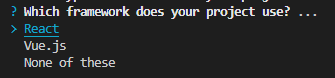
타입스크립트를 사용하고 있냐고 묻는다. No를 택했다.

어디에서 코드가 실행되냐 묻는다. Browser를 택했다.

최초 ESLint 스타일을 추천해준다고 한다. 인기있는 스타일을 골라보자.

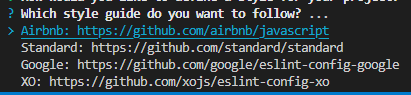
ESLint config 파일의 확장자를 묻는다. .js를 선택하겠다.

목록의 패키지들이 설치에 필요하다고 한다. 같이 설치해주자.

원하는 패키지 관리자를 선택하자.
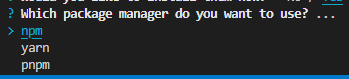
.eslintrc.js 파일이 생성되었다면 명령어를 입력해보자.
$ npx eslint test.js
eslintrc.js에서 정의한 규칙에 어긋난 내용들이 출력된다.
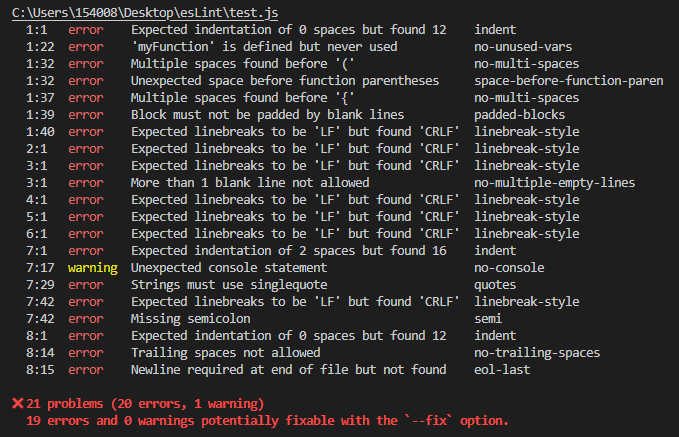
이번엔 명령어에 --fix 옵션을 부여하자.
$ npx eslint test.js --fix
코드가 강제로 수정되었다!!
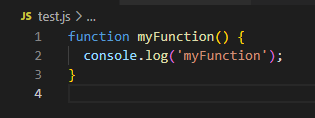
하지만 매번 커맨드로 이슈를 확인하고 수정하기에는 너무 번거롭다.
그래서 파일이 저장될 때마다 ESLint가 자동으로 실행되게 할 것인데,
VSCode의 Extensions를 사용하면 가능하다.
ESLint를 설치해주자.
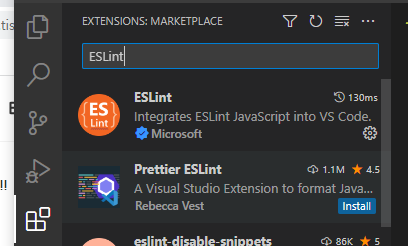
그리고 수정했던 파일을 다시 오류가 나게 되돌린다면. 이제 이슈들을 UI으로 확인할 수 있다.
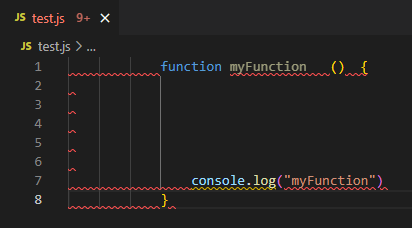
이제, 파일을 저장하면 포맷팅까지 적용이 되게 해보겠다.
Settings를 켠 다음 "editor format on save"를 검색하여 Format On Save 항목을 체크해주자.
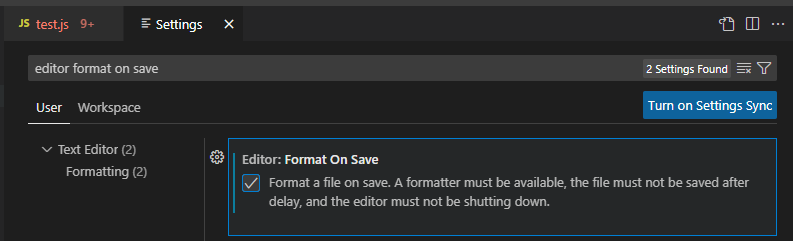
두 번째로 "editor code action on save"를 검색한 뒤에 Edit in settings.json을 클릭한다.
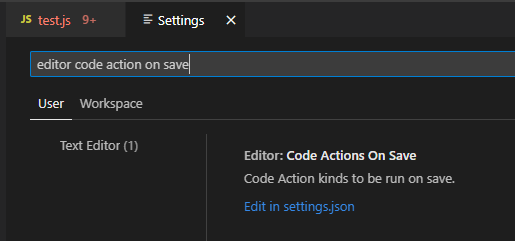
이동된 파일에서 아래 항목을 추가한다.
"editor.codeActionsOnSave": {
"source.fixAll": true
}
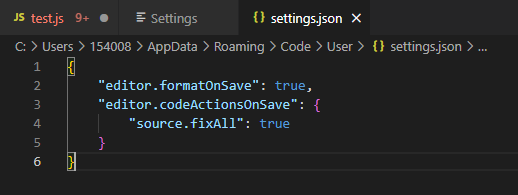
마지막으로, default formatter를 검색하여 Default Formatter 항목을 ESLint로 정의한다.
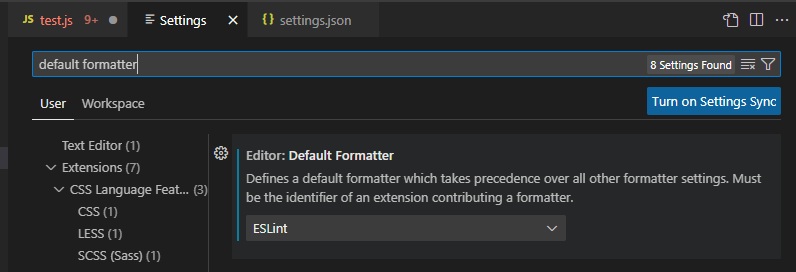
이제 파일을 저장한다면 자동으로 코드가 수정된다.
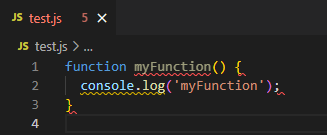
하지만 나의 경우 no-unused-vars, linebreak-style rule 이슈가 발생했는데,
이슈 코드를 강제로 변환시키지 않기 때문에 다른 방향으로 해결해주어야 한다.
마우스 커서를 빨간 라인에 올리면 노출되는 링크로 접속하면 해결방법에 대해 나와있다.
.eslintrc.js 파일의 rules 프로퍼티에 이슈를 무시하는 설정을 추가해주겠다.
rules: {
'no-unused-vars': 0,
'linebreak-style': 0,
},
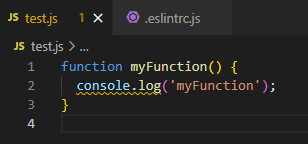
지금까지 Linter를 적용해봤다.
그렇다면 Formatter란 무엇인가?
Formatter로 대표적으로 사용되는 Prettier에서는 다음과 같이 정의한다.
Prettier vs. Linters
How does it compare to ESLint/TSLint/stylelint, etc.?Linters have two categories of rules:Formatting rules: eg: max-len, no-mixed-spaces-and-tabs, keyword-spacing, comma-style…Prettier alleviates the need for this whole category of rules! Prettier is going to reprint the entire program from scratch in a consistent way, so it’s not possible for the programmer to make a mistake there anymore :)Code-quality rules: eg no-unused-vars, no-extra-bind, no-implicit-globals, prefer-promise-reject-errors…Prettier does nothing to help with those kind of rules. They are also the most important ones provided by linters as they are likely to catch real bugs with your code!In other words, use Prettier for formatting and linters for catching bugs!
https://prettier.io/docs/en/comparison.html
로직적인 내용의 버그를 잡기 위해서는 Linter를 사용하고, 포맷팅 관련된 내용은 Formatter를 사용하는 것이 좋다고 한다.
지금까지 했던 내용에 prettier를 추가로 적용해보자.
명령어를 통해 prettier를 설치한다.
$ npm uninstall --save-dev eslint-config-prettier
.prettierrc.js 파일과 테스트 해보기 위한 옵션들을 추가해보자.
module.exports = {
tabWidth: 16,
}
.eslintrc.js 파일의 extends에 prettier를 추가한다.
module.exports = {
...,
extends: [
'plugin:react/recommended',
'airbnb',
'prettier'
],
...
};
VSCode extensions에서 prettier를 설치한다.
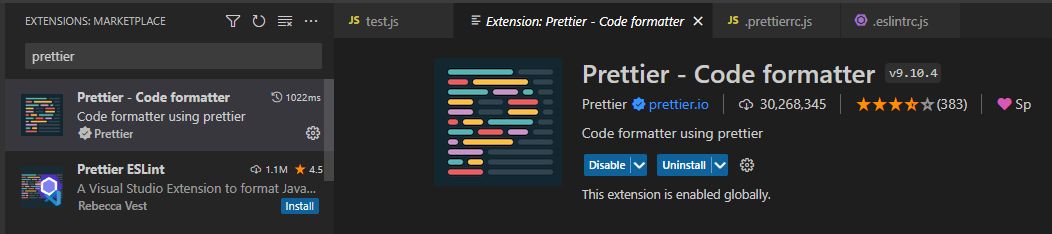
Settings의 Default Formatter 항목을 Prettier로 설정한다.
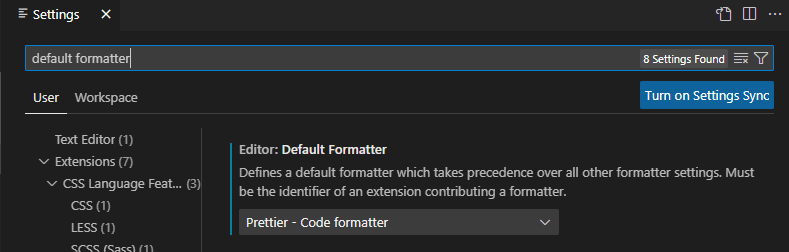
이제 test.js 파일을 저장하면 .prettierrc.js에서 설정한 것 처럼
들여쓰기가 16으로 설정된 상태로 코드가 수정이 된다.
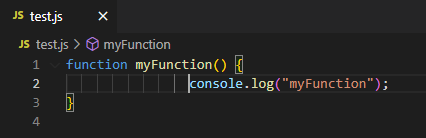
참조:
https://www.npmjs.com/package/eslint
eslint
An AST-based pattern checker for JavaScript.. Latest version: 8.36.0, last published: 6 days ago. Start using eslint in your project by running `npm i eslint`. There are 20266 other projects in the npm registry using eslint.
www.npmjs.com
https://prettier.io/docs/en/install.html
Prettier · Opinionated Code Formatter
Opinionated Code Formatter
prettier.io
https://github.com/prettier/eslint-config-prettier#installation
GitHub - prettier/eslint-config-prettier: Turns off all rules that are unnecessary or might conflict with Prettier.
Turns off all rules that are unnecessary or might conflict with Prettier. - GitHub - prettier/eslint-config-prettier: Turns off all rules that are unnecessary or might conflict with Prettier.
github.com
https://prettier.io/docs/en/comparison.html
Prettier · Opinionated Code Formatter
Opinionated Code Formatter
prettier.io
https://prettier.io/docs/en/configuration.html
Prettier · Opinionated Code Formatter
Opinionated Code Formatter
prettier.io
'기타 공부' 카테고리의 다른 글
[CSS] HTML table element 행렬 pivot하기 (0) | 2023.10.11 |
---|---|
[Git] 실무에서 자주 사용되는 commands (0) | 2023.02.08 |
MSA 한번에 이해하기 (0) | 2021.11.16 |
나중에 보려고 스크랩 해둔 CORS 문제 (0) | 2020.07.07 |
babel, webpack (0) | 2020.07.07 |